In the ever-evolving landscape of computer science and software development, Java remains an indomitable force. Renowned for its versatility, stability, and platform independence, Java has shaped the digital world for decades. This article delves into the intricacies of Java programming, unveiling its fundamental concepts, exploring its unique features, and showcasing its widespread applications. Whether you are an aspiring developer seeking to enter the realm of coding or an accomplished programmer looking to broaden your expertise, join us on this enthralling journey as we unravel the wonders of Java.
Table of Contents
I. The Foundation of Java: Object-Oriented Paradigm
Java’s foundation lies in its object-oriented paradigm, which is the bedrock of modern programming. By embracing objects and classes, Java empowers developers to create reusable, modular, and scalable code. Object-oriented programming (OOP) embodies the principles of encapsulation, inheritance, polymorphism, and abstraction, enabling programmers to model real-world entities in a structured manner.
A. Understanding Objects and Classes
In Java, objects represent tangible entities, while classes serve as blueprints for creating these objects. The interplay of classes and objects fosters code re-usability and promotes the efficient organization of software components. Each object encapsulates its state (data) and behavior (methods), and through careful design, developers can ensure that objects interact with each other seamlessly.
When defining classes, developers determine the attributes and behaviors shared by the objects of that class. Encapsulation, an essential aspect of OOP, shields the internal details of a class from the external world, ensuring that objects interact only through well-defined interfaces. This approach enhances code maintainability and reduces the risk of unintended side effects.
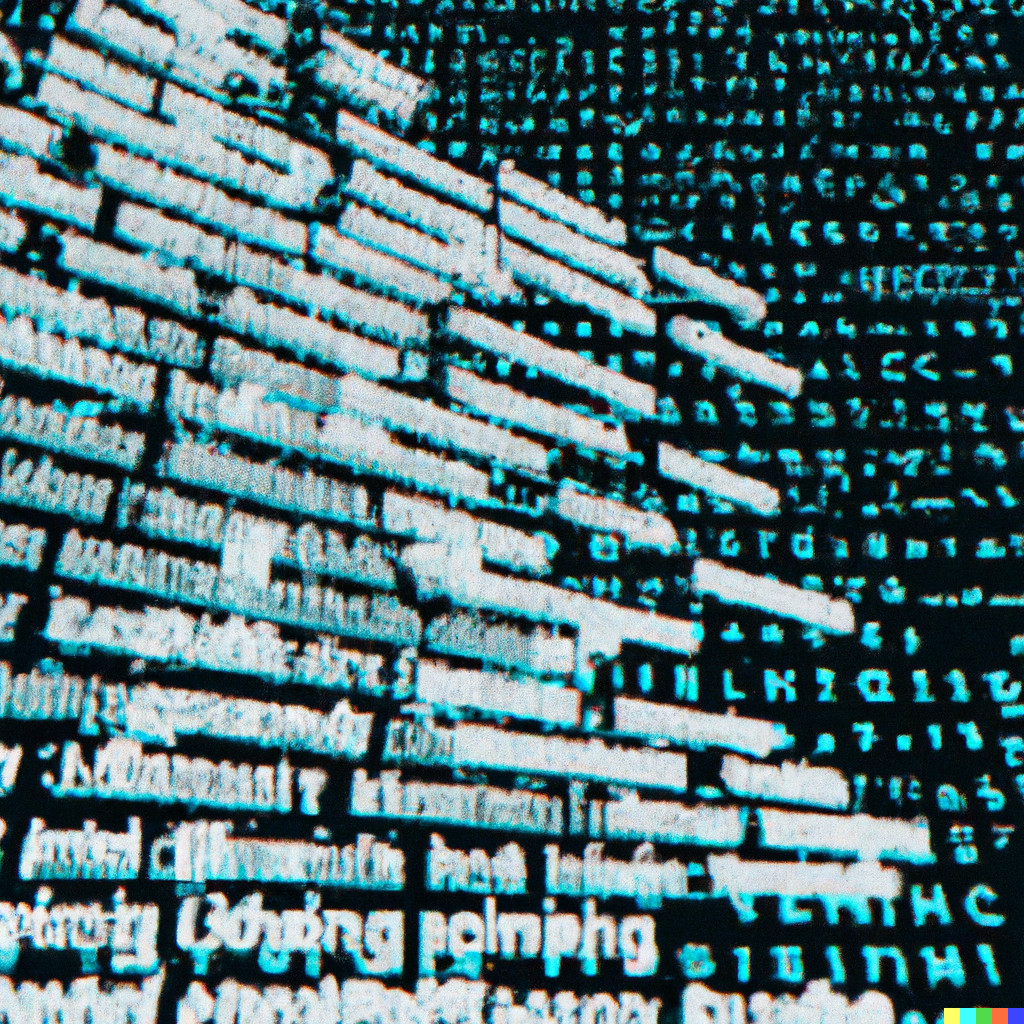
B. Mastering Inheritance and Polymorphism
Inheritance, a core tenet of OOP, allows classes to derive properties and behaviors from other classes. By creating a new class from an existing one, developers can reuse the features of the parent class and extend its functionality. This hierarchical relationship between classes fosters code organization and enhances code readability.
Polymorphism, a Greek word meaning “many forms,” enables objects to assume multiple forms and behave differently based on their context. The two primary types of polymorphism in Java are compile-time (method overloading) and runtime (method overriding). Method overloading allows multiple methods with the same name but different parameter lists, while method overriding allows a subclass to provide a specific implementation of a method already defined in its superclass.
C. Embracing Abstraction for Elegance
Abstraction empowers programmers to focus on essential attributes while hiding intricate implementation details. Java’s abstraction mechanisms facilitate the creation of clear and concise code, elevating the readability and maintainability of projects. Abstract classes and interfaces play a pivotal role in achieving abstraction.
Abstract classes act as a blueprint for other classes, allowing them to define common attributes and methods. However, abstract classes cannot be instantiated directly; they serve as a foundation for subclasses to extend and implement their specific functionalities. Interfaces, on the other hand, define contracts that classes must adhere to, specifying method signatures without implementation details. A class can implement multiple interfaces, enabling developers to achieve multiple inheritances through interfaces.
Implementing abstraction encourages a modular approach, where each class focuses on a specific task, making the codebase more maintainable and adaptable to changes. Moreover, abstraction plays a crucial role in designing frameworks and libraries, as it allows developers to provide generic solutions that can be customized by other programmers.
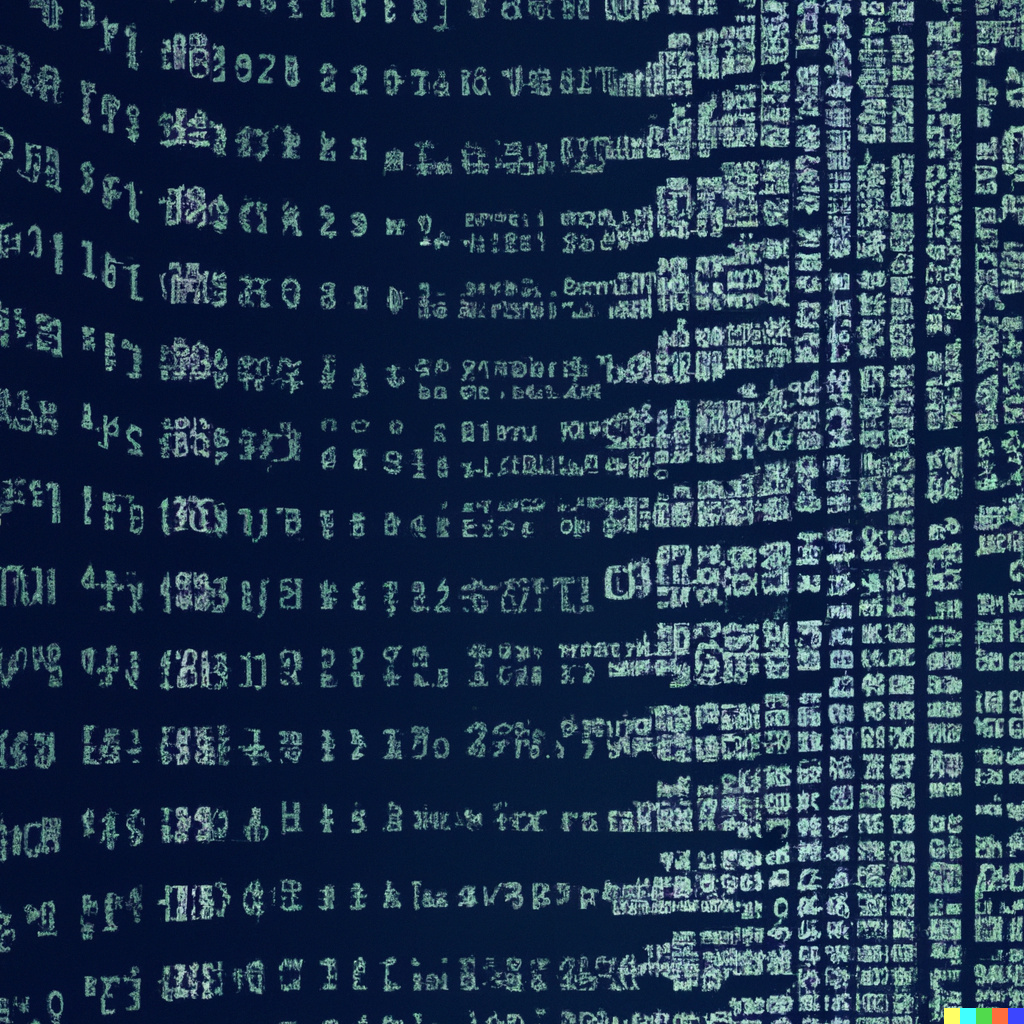
II. Harnessing the Power of Java Libraries and APIs
The Java ecosystem boasts a rich assortment of libraries and Application Programming Interfaces (APIs) that expedite development and extend the language’s capabilities. These libraries cover a vast array of domains, ranging from GUI development and database connectivity to networking and cryptography.
A. Streamlining Development with Standard Libraries
Java’s standard library encompasses a treasure trove of pre-built classes and methods, carefully curated to handle common programming tasks. From data structures like arrays, lists, and maps to input/output operations and multi-threading, the standard library provides essential tools for developers to streamline their projects.
One of the most prominent features of the standard library is the Collections Framework, which offers a comprehensive set of interfaces and classes for handling collections of objects, such as lists, sets, and queues. The Collections Framework employs generics, a feature introduced in Java 5, to ensure type safety and enhance code readability.
Additionally, the standard library includes utilities for handling dates and times, managing exceptions, working with regular expressions, and more. By leveraging these ready-made components, developers can focus on implementing business logic rather than reinventing the wheel, ultimately accelerating development and improving code quality.
B. Exploring Third-party Libraries
Beyond the standard library, Java developers can tap into an extensive selection of third-party libraries tailored for specific needs. These libraries, often open-source and actively maintained by the community, enhance functionality and offer solutions to intricate challenges.
For instance, Apache Commons provides a plethora of reusable Java components that address common programming tasks, such as string manipulation, mathematical operations, and file management. Google Guava, on the other hand, extends the Collections Framework with additional utilities, functional programming support, and caching mechanisms.
Libraries like JUnit facilitate automated testing, ensuring robustness and reliability in software projects. Similarly, frameworks like Hibernate enable seamless interaction with databases through Object-Relational Mapping (ORM), abstracting away the complexities of SQL queries and database management.
By incorporating third-party libraries into their projects, developers can capitalize on the collective expertise of the Java community, saving time and effort while delivering high-quality applications.
C. Interacting with Java APIs
APIs provide a structured interface for communication between different software components. Java’s APIs facilitate interaction with system functionalities, databases, web services, and more. Understanding how to leverage these APIs effectively is crucial for unlocking the full potential of Java programming.
Java’s standard API includes a diverse range of packages, from java.io for input/output operations to java.net for networking. The java.sql package provides the necessary classes and interfaces for working with relational databases, while the javax.swing package allows developers to create Graphical User Interfaces (GUIs) for desktop applications.
Moreover, Java has embraced the world of web services with the Java API for RESTful Web Services (JAX-RS) and Java API for XML Web Services (JAX-WS). These APIs facilitate the creation and consumption of web services, enabling seamless communication between different software systems over the internet.
In recent years, Java’s APIs have evolved to accommodate cloud computing and microservices architecture. APIs like Jakarta Persistence, previously known as Java Persistence API (JPA), and Java API for JSON Processing (JSON-P) cater to these modern paradigms, enabling developers to build scalable and flexible applications.
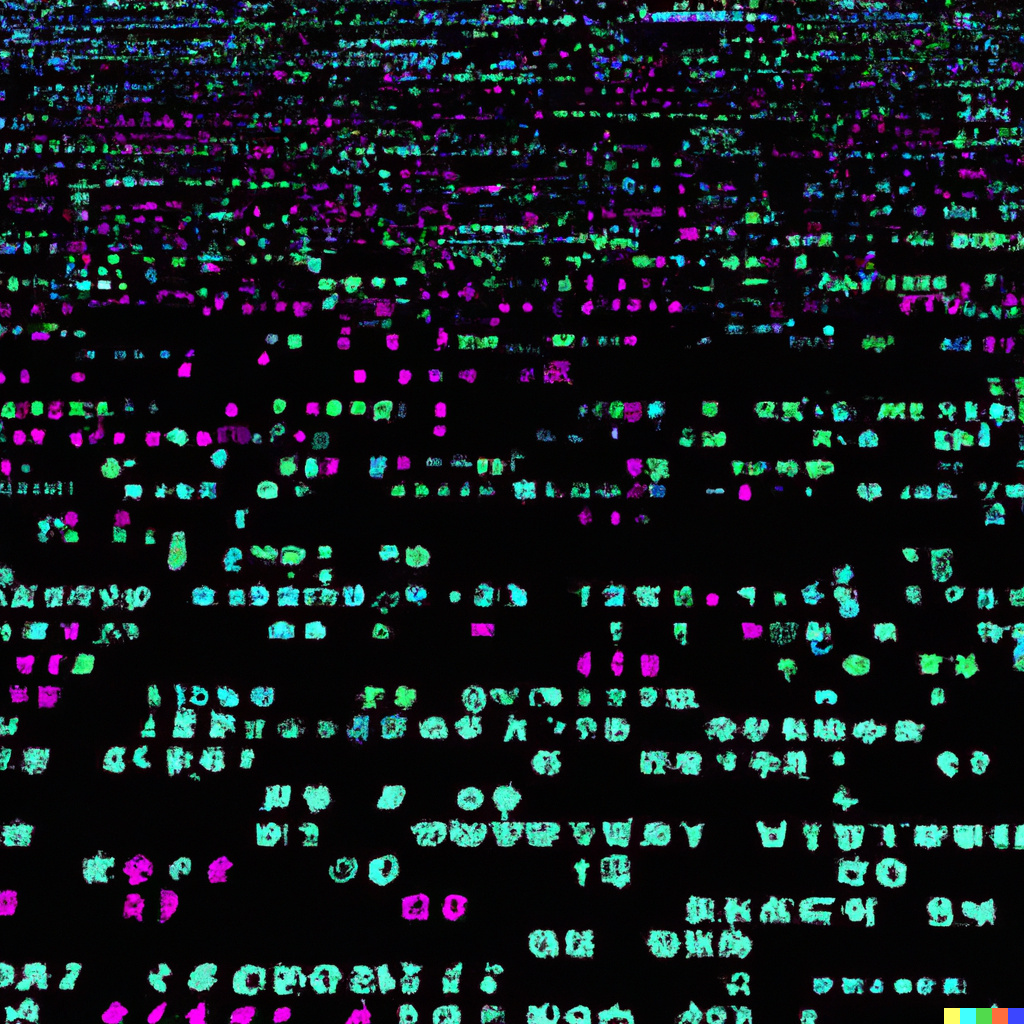
III. Java in Practice: Real-world Applications and Future Prospects
Java’s adaptability has led to its widespread adoption in a myriad of domains, from web development and mobile applications to enterprise solutions and embedded systems. As technology continues to evolve, Java remains steadfast and shows promising prospects in shaping the future of software development.
A. Java in the Web Development Landscape
Java’s presence in web development is pervasive, with frameworks like Spring and JavaServer Faces (JSF) empowering developers to build feature-rich web applications. The Spring Framework, in particular, offers a comprehensive suite of tools and modules for building enterprise-level applications. Its dependency injection mechanism, coupled with robust support for various architectural patterns, enhances code maintainability and promotes best practices in development.
JSF, on the other hand, simplifies the creation of user interfaces by providing a component-based model, allowing developers to assemble complex web pages with reusable and customizable components. By combining JavaServer Faces with JavaServer Pages (JSP) and Enterprise JavaBeans (EJB), developers can build enterprise-grade applications catering to diverse business requirements.
B. Java in Mobile App Development
With the rise of Android, Java has become the de facto language for developing mobile applications. Java, alongside the Android Software Development Kit (SDK), enables developers to create engaging and feature-rich applications for smartphones and tablets.
Android’s robust architecture, coupled with Java’s versatility, allows developers to harness hardware capabilities and create innovative applications that seamlessly integrate with the mobile ecosystem. From multimedia-rich applications to location-based services and augmented reality experiences, Java remains at the heart of Android development.
The advent of Kotlin, a language designed for Android development, has also influenced the mobile development landscape. While Kotlin provides improved syntax and enhanced safety features, Java continues to be a strong contender for building Android applications, especially for those developers who already possess Java expertise.
C. Java for Scalable Enterprise Solutions
Java’s robustness and scalability make it an ideal choice for crafting large-scale enterprise solutions. From Customer Relationship Management (CRM) systems to Big Data processing, Java powers a wide spectrum of mission-critical applications.
In the realm of server-side development, Java’s versatility is showcased through frameworks like JavaServer Pages (JSP), Java Servlets, and the aforementioned Spring Framework. These technologies allow developers to build scalable web applications and RESTful services, capable of handling a high volume of concurrent requests.
Enterprise JavaBeans (EJBs) play a crucial role in developing distributed applications. EJBs provide a component-based model that simplifies the development of distributed, transactional, and secure applications for Java Enterprise Edition (EE) environments.
Moreover, Java’s integration with Big Data technologies, such as Apache Hadoop and Apache Spark, reinforces its position as a potent tool for processing and analyzing vast datasets. Java’s ability to parallelize computations and its support for distributed computing enable seamless data processing in large-scale, data-intensive applications.
Java programming stands as a timeless art form, with its object-oriented paradigm, powerful libraries, and diverse applications. In this article, we have traversed the many facets of Java’s capabilities, from the intricacies of object-oriented programming to the vast landscape of libraries, APIs, and real-world applications.
The journey into Java programming continues to be an enriching one, as its versatility and adaptability keep it relevant in the rapidly evolving world of technology. Whether you are a seasoned programmer or an aspiring enthusiast, mastering Java opens doors to an ever-expanding universe of opportunities in the realm of computer science and beyond. So, pick up your IDE, embrace the elegance of Java, and let your imagination and code intertwine to create innovative solutions for the world of tomorrow.